Why you need a custom context provider
Naked Context providers can be a killer for performance. In this article, we will explore why Custom Context providers have a positive impact and should be the default when using the Context API.
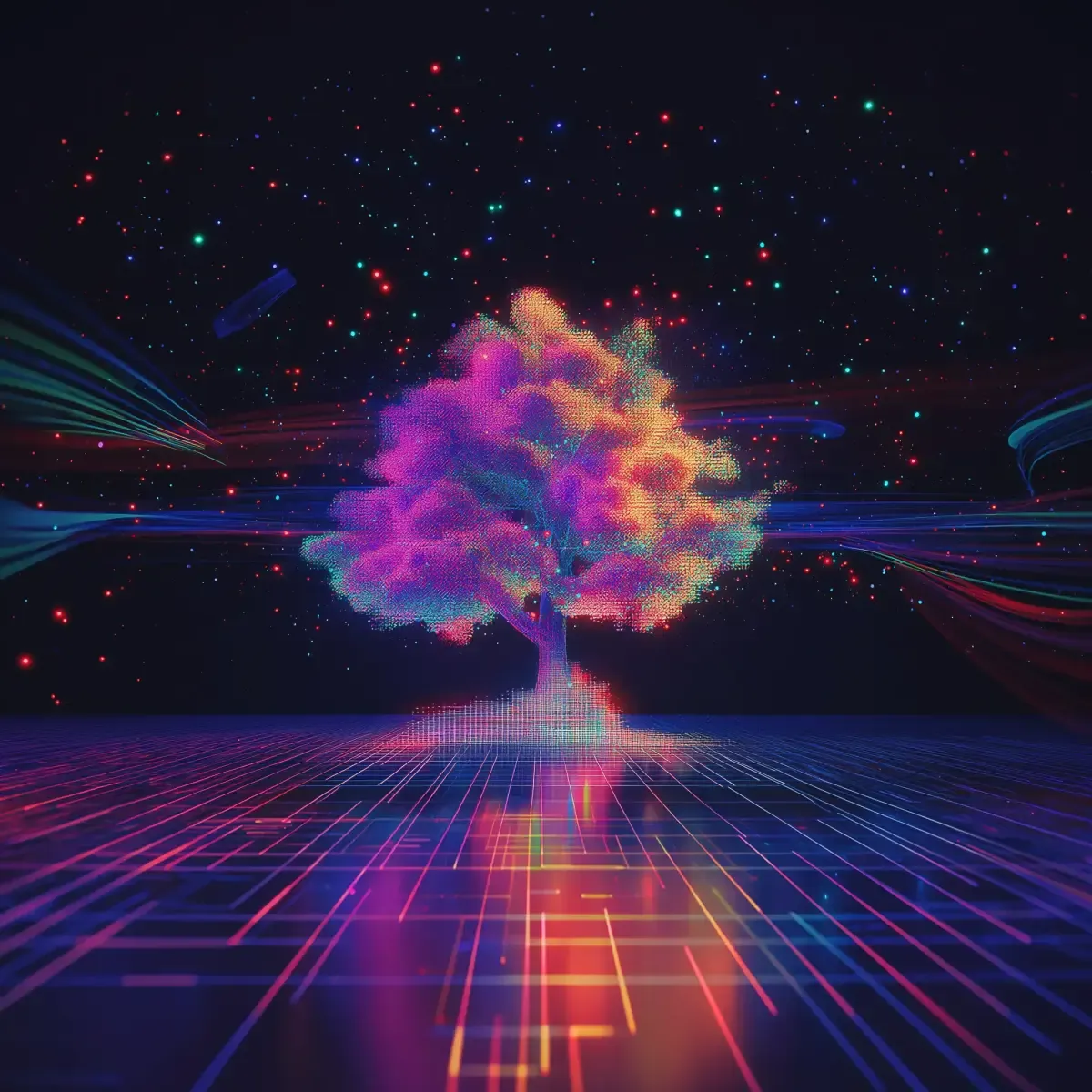
Part of my missions involve auditing existing React applications and helping teams fix performance issues they encounter. One common problem I’ve seen across multiple projects is inefficient use of the Context API which leads to unnecessary re-renders. This article addresses one of the most impactful patterns I recommend: implementing custom context providers.
By adopting this pattern, teams can improve the performance of their applications, especially in complex UIs with deeply nested component trees. Let’s explore why this approach matters and how to implement it.
Contents
- What is a Custom Context Provider?
- The Problem with Context and Re-renders
- Why do we have these unnecessary re-renders?
- Using a Custom Context Provider (preferred)
- Another solution: Memoizing Context Provider children
- Conclusion
- References
What is a Custom Context Provider?
A custom context provider is a dedicated component that encapsulates the state
management logic for a specific context in React. Unlike a “naked” context
provider (where you directly use <Context.Provider>
in your component tree), a
custom provider separates the concern of state management from your component
hierarchy.
Naked Context Provider:
// State and context usage mixed in the same componentfunction App() { const [count, setCount] = useState(0);
return ( <CounterContext.Provider value={count}> {/* ... other components ... */} </CounterContext.Provider> );}
The “naked” is a wording that I coined: the Provider is not “dressed”, it lacks a function to wrap it and keep it warm.
Custom Context Provider:
// Dedicated provider componentfunction CounterProvider({ children }) { const [count, setCount] = useState(0);
return ( <CounterContext.Provider value={count}>{children}</CounterContext.Provider> );}
function App() { return <CounterProvider>{/* ... other components ... */}</CounterProvider>;}
The custom approach provides better separation of concerns, improved performance, and a cleaner component tree. Let’s explore why this has an impact on performance.
The Problem with Context and Re-renders
The ReactJS doc states1:
"All consumers that are descendants of a Provider will re-render whenever the Provider’s value prop changes. The propagation from Provider to its descendant consumers (including .contextType and useContext) is not subject to the shouldComponentUpdate method, so the consumer is updated even when an ancestor component skips an update.
"
In other words, a component that consumes a context will be re-rendered when the context value changes.
The thing is, if the state of a component changes, it will re-render and it will re-render its children.
So let’s have a look at the “naked” context provider usage below:
Naked Context Provider
Click the increment button and observe which components re-render
import React, { createContext } from "react";import { Box } from "../common/Box";
const CounterContext = createContext<{ count: number; increment: () => void;}>({ count: 0, increment: () => {},});
function ContextSetter() { const { count, increment } = React.useContext(CounterContext);
return ( <Box name="ContextSetter"> <button type="button" onClick={increment} className="button-fancy"> Increment Counter: {count} </button> </Box> );}
function UnrelatedComponent() { return ( <Box name="Unrelated"> <div className="text-lg"> This component re-renders when the context value changes. </div> </Box> );}
export function App() { const [count, setCount] = React.useState(0); const increment = () => setCount((c) => c + 1);
return ( <Box name="App"> <CounterContext.Provider value={{ count, increment }}> <div className="flex flex-col space-y-4"> <ContextSetter /> <UnrelatedComponent /> </div> </CounterContext.Provider> </Box> );}
If you click the “Increment” button, the count
state will be updated and all
the components will be re-rendered, even the ones that don’t use the context.
Indeed, when you increment the counter the count
state is updated and the
component is re-rendered as well as all its children. If the update of our
context is triggered, the whole App
component is re-rendered.
Now let’s see how a custom context provider implementation would behave:
Custom Context Provider
Click the increment button and observe which components re-render
import React, { createContext, useContext, useState } from "react";import { Box } from "../common/Box";
const CounterContext = createContext<{ count: number; increment: () => void;}>({ count: 0, increment: () => {},});
function CounterButton() { const { count, increment } = useContext(CounterContext);
return ( <Box name="CounterButton"> <button onClick={increment} className="button-fancy"> Increment Counter : {count} </button> </Box> );}
function UnrelatedComponent() { return ( <Box name="Unrelated"> <div className="text-lg"> This component DOES NOT re-renders when the context value changes. </div> </Box> );}
export function CounterProvider({ children }: { children: React.ReactNode }) { const [count, setCount] = useState(0);
const value = { count, increment: () => setCount((c) => c + 1), };
return ( <CounterContext.Provider value={value}>{children}</CounterContext.Provider> );}
export function CustomProviderApp() { return ( <Box name="App"> <CounterProvider> <div className="flex flex-col space-y-4"> <CounterButton /> <UnrelatedComponent /> </div> </CounterProvider> </Box> );}
Notice how with the custom provider implementation:
- Only the components that actually use the context value re-render
- The unrelated component does not re-render even though it’s a child of the Provider that changes
This is achieved by creating a dedicated provider component that memoizes the context value:
// Create a dedicated provider componentfunction CounterProvider({ children }) { const [count, setCount] = useState(0);
// Memoize the value to prevent unnecessary re-renders const value = useMemo( () => ({ count, increment: () => setCount((c) => c + 1), }), [count], );
return ( <CounterContext.Provider value={value}>{children}</CounterContext.Provider> );}
// Usage in your appfunction App() { return ( <CounterProvider> <YourComponents /> </CounterProvider> );}
With this approach, only components that actually consume the context will re-render when the context value changes.
Why do we have these unnecessary re-renders?
When using a naked context provider, the context value is defined in the “parent” component.
Let’s look at what happens in our first example:
- User clicks “Increment” button
count
state updatesApp
component re-renders- A new
value
object is created:{ count, increment }
CounterContext.Provider
receives a new value reference
Which components re-render?
Rule 1: if a component re-renders, all its children re-render
Rule
2: if a context value changes, all consumers re-render
Therefore here we don’t care about Rule 2. As App
re-renders, all its children
re-render. This includes Counter
and UnrelatedComponent
.
This may cause a significant performance issue regarding unnecessary re-renders. Components that don’t actually use the context values still re-render because they’re children of the provider component.
It can be illustrated as below:
The whole App and all its children re-render when the context value changes.
Using a Custom Context Provider (preferred)
As showcased above, the Custom Context Provider approach solves this issue by only re-rendering what is necessary: the provider and the consumer, like shown on the schema below.
Why this?
function CounterProvider({ children }) { const [count, setCount] = useState(0);
// Memoize the value to prevent unnecessary re-renders const value = useMemo( () => ({ count, increment: () => setCount((c) => c + 1), }), [count], );
return ( <CounterContext.Provider value={value}>
{children} // <-- Yes this one </CounterContext.Provider> );}
This is what we call composition (or the
children prop pattern).
When CounterProvider
re-renders, we could think that children
elements are
rendered too. This is not the case, the children
reference stays stable in
this case. Therefore React does a clever trick and keeps the element as is. If
the context value changes though, it will trigger the re-render of the
consumers.
Another solution: Memoizing Context Provider children
While this approach can work, it adds complexity to your component structure and requires careful management of memoization dependencies.
With this approach we memoize the children of the context provider using
React.memo
or useMemo
:
const App = () => { const [count, setCount] = useState(0);
const value = { count, increment: () => setCount((c) => c + 1), };
// Memoize the children that don't need context updates const memoizedDeepComponent = useMemo(() => <DeepComponent />, []);
return ( <CounterContext.Provider value={value}> <div> <h1>Counter: {count}</h1> <Counter /> {memoizedDeepComponent} </div> </CounterContext.Provider> );};
This is strictly equivalent to the custom context provider approach, but it requires to be more cautious on the dependencies of memoization.
Be careful with this approach as it can lead to bugs if you forget to update memoization dependencies when your component logic changes.
Conclusion
When using React Context with state, always consider the performance implications of your implementation. A “naked” context provider will mostly lead to bad performance due to unnecessary re-renders. Instead, use a custom context provider.
There are more points to Context optimization, like context value memoization, but that will be the topic of another article.
References
This article was drafted in 2022, therefore it still points to the old React documentation
- Kent C. Dodds Article: How to use React Context effectively
- React Documentation: Optimizing Performance
- Dan Abramov’s Overreacted: Before You memo()
Footnotes
-
2022-05-09 - Context Provider ↩